When working with Odoo, understanding relational fields like Many2One, One2Many, and Many2Many is essential for creating efficient data models. These fields allow us to define relationships between different models, ensuring data consistency and logical connections. In this blog, we’ll explain these concepts in simple terms with practical examples.
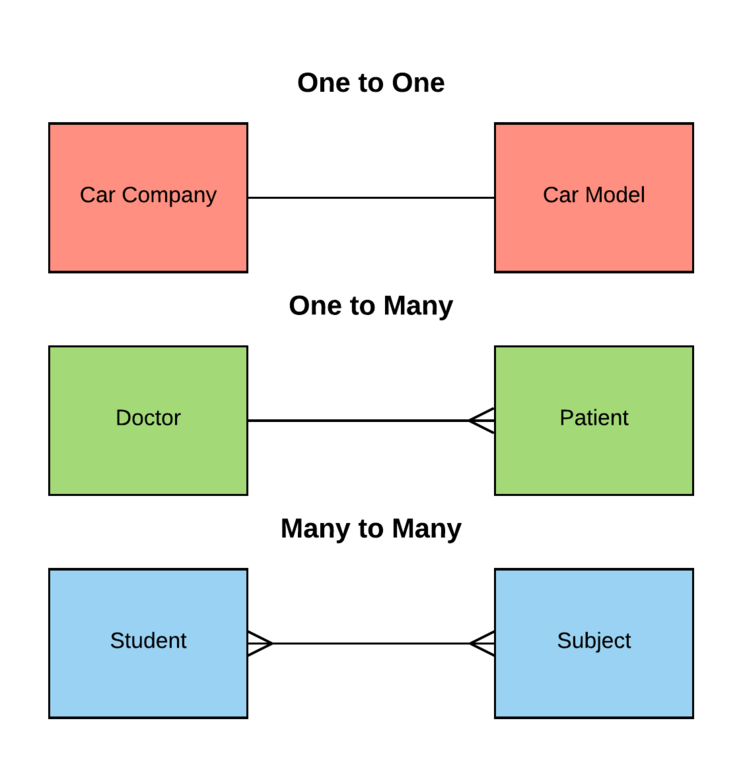
—————————————————————————-
1. Many2One Relationship
A Many2One field creates a “many-to-one” relationship, meaning multiple records in one model can be linked to a single record in another model.
Example:
Imagine you’re building a “Hospital Management System.” Each patient is assigned to a specific doctor.
Models:
- Doctor
- Patient
class Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_id = fields.Many2One('hospital.doctor', string="Assigned Doctor")
Here:
doctor_id
in thePatient
model refers to a record in theDoctor
model.- Multiple patients can have the same doctor.
How It Looks in Odoo:
In the form view for a patient, you’ll see a dropdown to select a doctor.
2. One2Many Relationship:
A One2Many field defines a “one-to-many” relationship, where one record in a model is associated with multiple records in another model. This is the inverse of Many2One.
Example:
Let’s say a doctor can have multiple patients.
class Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
patient_ids = fields.One2Many('hospital.patient', 'doctor_id', string="Patients")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_id = fields.Many2One('hospital.doctor', string="Assigned Doctor")
Here:
patient_ids
in theDoctor
model is a list of all patients assigned to the doctor.
How It Looks in Odoo:
In the form view for a doctor, you’ll see a list of their patients.
3. Many2Many Relationship:
A Many2Many field creates a “many-to-many” relationship, meaning multiple records in one model can be linked to multiple records in another model.
Example:
Suppose patients can be assigned to multiple doctors for collaborative care.
class Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
patient_ids = fields.Many2Many('hospital.patient', string="Patients")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_ids = fields.Many2Many('hospital.doctor', string="Doctors")
Here:
patient_ids
in theDoctor
model lists all patients for that doctor.doctor_ids
in thePatient
model lists all doctors for that patient.
How It Looks in Odoo:
Both doctor and patient forms will display many-to-many widgets to select multiple records.
Understanding Many2One, One2Many, and Many2Many Relationships in Odoo
When working with Odoo, understanding relational fields like Many2One, One2Many, and Many2Many is essential for creating efficient data models. These fields allow us to define relationships between different models, ensuring data consistency and logical connections. In this blog, we’ll explain these concepts in simple terms with practical examples.
1. Many2One Relationship
A Many2One field creates a “many-to-one” relationship, meaning multiple records in one model can be linked to a single record in another model.
Example:
Imagine you’re building a “Hospital Management System.” Each patient is assigned to a specific doctor.
Models:
- Doctor
- Patient
Code:
pythonCopy codeclass Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_id = fields.Many2One('hospital.doctor', string="Assigned Doctor")
Here:
doctor_id
in thePatient
model refers to a record in theDoctor
model.- Multiple patients can have the same doctor.
How It Looks in Odoo:
In the form view for a patient, you’ll see a dropdown to select a doctor.
2. One2Many Relationship
A One2Many field defines a “one-to-many” relationship, where one record in a model is associated with multiple records in another model. This is the inverse of Many2One.
Example:
Let’s say a doctor can have multiple patients.
Code:
pythonCopy codeclass Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
patient_ids = fields.One2Many('hospital.patient', 'doctor_id', string="Patients")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_id = fields.Many2One('hospital.doctor', string="Assigned Doctor")
Here:
patient_ids
in theDoctor
model is a list of all patients assigned to the doctor.
How It Looks in Odoo:
In the form view for a doctor, you’ll see a list of their patients.
3. Many2Many Relationship
A Many2Many field creates a “many-to-many” relationship, meaning multiple records in one model can be linked to multiple records in another model.
Example:
Suppose patients can be assigned to multiple doctors for collaborative care.
Code:
pythonCopy codeclass Doctor(models.Model):
_name = 'hospital.doctor'
name = fields.Char(string="Doctor Name")
patient_ids = fields.Many2Many('hospital.patient', string="Patients")
class Patient(models.Model):
_name = 'hospital.patient'
name = fields.Char(string="Patient Name")
doctor_ids = fields.Many2Many('hospital.doctor', string="Doctors")
Here:
patient_ids
in theDoctor
model lists all patients for that doctor.doctor_ids
in thePatient
model lists all doctors for that patient.
How It Looks in Odoo:
Both doctor and patient forms will display many-to-many widgets to select multiple records.
Key Differences
Field | Relationship Type | Example |
Many2One | Many to One | Many patients → One doctor |
Many2Many | One to Many | One doctor → Many patients |
Many2Many | Many to Many | Many doctors ↔ Many patients |
When to Use These Fields?
- Use Many2One when a record in one model must link to a single record in another.
- Use One2Many to display or manage a list of related records.
- Use Many2Many when records in both models can have multiple links to each other.